Code Issues Pull requests. Although there are different methods to encrypt and decrypt messages, we'll focus on a linear algebra-based cipher, the Hill cipher, which uses a matrix as a cipher to encode a message or an image, and it's extremely difficult to break when a large matrix is used. The Hill cipher, like most classical ciphers from the pre-computer era, was traditionally used to only encrypt letters: that is, the valid inputs would consist only of the 26 letters from A to Z (and, in some variants, possibly a few extra symbols to make the alphabet size a prime number). Hill cipher is a block cipher method and repetition won’t be cause weakness. Still, I prefer to append beginning of the message instead of repeating characters. BTW, column number of my message and row number of my key are equal. The following code block won’t be run for this case. Playfair Cipher Program in C. In this program, we have entered the MONARCHY and the message as LEARNPROGRAMO and the final message is printed on the screen. #include #include #include int removerepeated (int size,int a ); int insertelementat (int position,int a ,int size); main int i,j,k,numstr 100. The program should handle keys and text of unequal length, and should capitalize everything and discard non-alphabetic characters. (If your program handles non-alphabetic characters in another way, make a note of it.) Related tasks Caesar cipher Rot-13 Substitution Cipher.
According to the definition in wikipedia, in classical cryptography, the Hill cipher is a polygraphic substitution cipher based on linear algebra. Invented by Lester S. Hill in 1929, it was the first polygraphic cipher in which it was practical (though barely) to operate on more than three symbols at once. The explanation of cipher, which is below the calculator, assumes an elementary knowledge of matrices.
Hill Cipher Program Source Codes
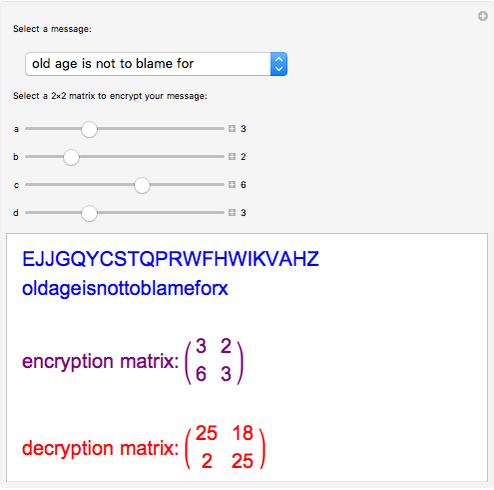
How does it work
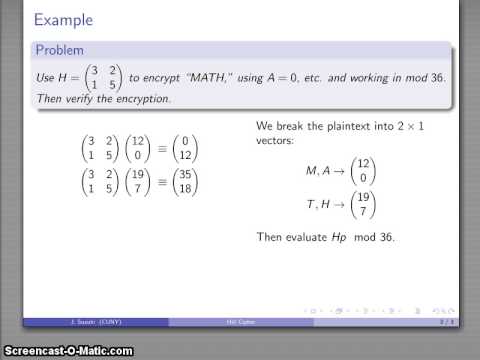
First, symbols of the used alphabet (alphabet as a set of symbols, for example, the alphabet in the above calculator includes space, comma, and dot symbols) are encoded with digits, for example, symbol's order number in the set. Then we choose a matrix of n x n size, which will be the cipher's key. Text is divided into blocks of size n, and each block forms a vector of size n. Each vector is multiplied by the key matrix of n x n. The result, vector of size n, is a block of encrypted text. Modular arithmetic is used; that is, all operations (addition, subtraction, and multiplication) are done in the ring of integers, where the modulus is m - the length of the alphabet. This allows us to force results to belong to the same alphabet.
Key is the matrix; however, it is convenient to use the key phrase, which is transformed into the digit representation and matrix. In order to create a n x n size matrix, keyphrase length should be square of an integer, i.e., 4, 9, 16.
Additional restrictions to the key are imposed by the need to decrypt encrypted text :)
And, for this to happen, we need to have a modular inverse of the key matrix in - ring of integers modulo m.
If source vector B is multiplied by matrix A to get vector C, then to restore vector B from vector C (decrypt text), one needs to multiply it by the modular inverse of the matrix.
Thus they have the following restrictions:
The determinant of the matrix should not be equal to zero, and, additionally, the determinant of the matrix should have a modular multiplicative inverse.
The formula imposes the latter
.
where the operation of multiplication substitutes the operation of division by the modular multiplicative inverse.
In order to have a modular multiplicative inverse, determinant and modulo (length of the alphabet) should be coprime integers, refer to Modular Multiplicative Inverse. In order to increase the probability of this, the alphabet is expanded, so its length becomes the prime integer. That is why the English alphabet in the calculator above is expanded with space, comma, and dot up to 29 symbols; 29 is a prime integer.
Hill Cipher Program Source Code Download
Not every key phrase is qualified to be the key; however, there are still more than enough.
In cryptography, a cipher (or cypher) is an algorithm for performing encryption or decryption—a series of well-defined steps that can be followed as a procedure. An alternative, less common term is encipherment. To encipher or encode is to convert information into cipher or code. In common parlance, “cipher” is synonymous with “code“, as they are both a set of steps that encrypt a message; however, the concepts are distinct in cryptography, especially classical cryptography.
Codes generally substitute different length strings of character in the output, while ciphers generally substitute the same number of characters as are input. There are exceptions and some cipher systems may use slightly more, or fewer, characters when output versus the number that was input.
Hill Cipher Program Source Code Example
In this post, we will discuss the Playfair Cipher. The Playfair cipher is a cryptographic technique that is used to encrypt/decrypt a message. The technique encrypts pairs of letters (bigrams or digrams), instead of single letters as in the simple substitution cipher and rather more complex Vigenère cipher systems then in use.
Working
The Playfair cipher uses a 5 by 5 table of letters. To generate the key, we will first fill the table row-wise with the letters of the key. We omit the repeating letters. After this, we fill the table with the remaining letters. We usually omit the letter i or j so that the number of letters in the table is 25. After the table is generated, we divide the message into the pairs of 2. Then for each pair, we look up the position of the letters in the table. Suppose the pair is XY and X is at position (x1,y1) and Y is at position (x2,y2), we choose the letters which are at position (x2,y1) and (x1,y2). If the pairs are (x,y1) and (x,y2), then we choose the letters at (x,y1+1) and (x,y2+1).
If the pairs are (x1,y) and (x2,y), then we choose the letters at (x1+1,y) and (x2+1,y).
For example, if the message is “helloworld” and the key is “test”. We will generate the following table:
The original message and the encrypted message will be:
We will use C++ to write this algorithm due to the standard template library support. Hence, we will write the program of the Playfair Cipher algorithm in C++, although, it’s very similar to C.
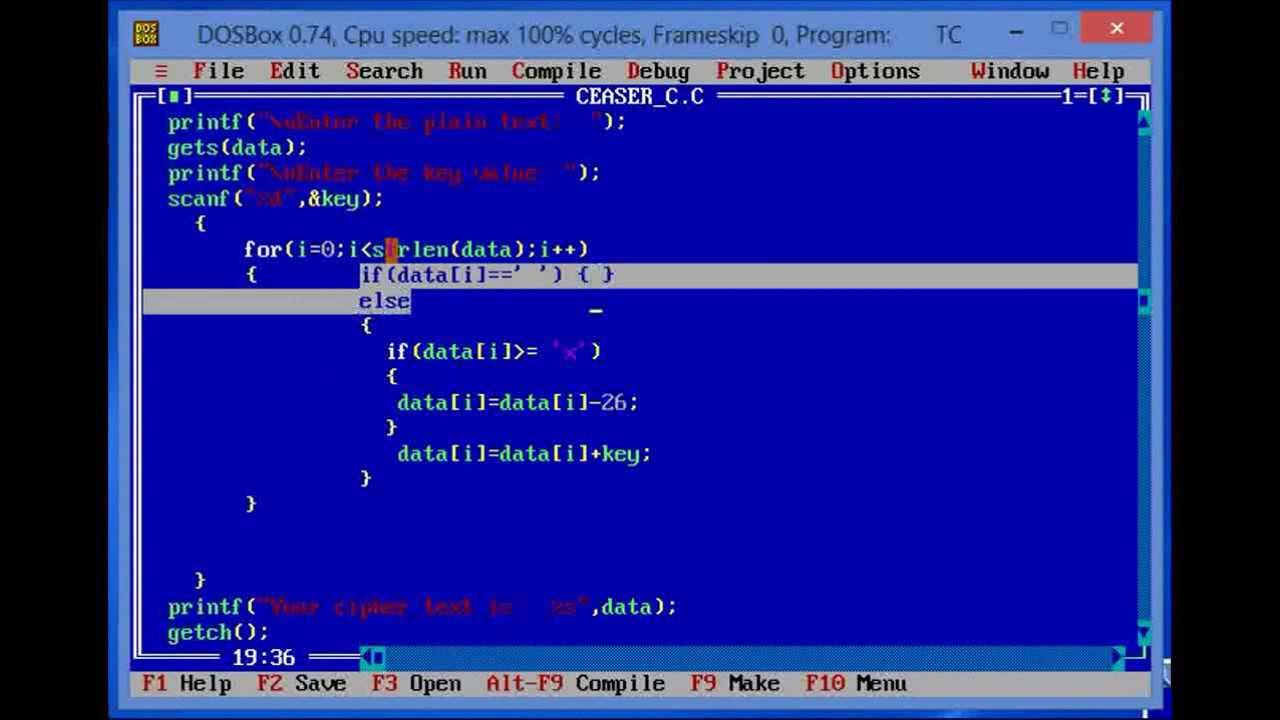
Encryption
INPUT:
line 1: message
line 2: key
Hill Cipher Program Source Code
OUTPUT:
line 1: Encrypted message
The following is the Playfair Cipher encryption algorithm program in C++.
OUTPUT:
Decryption
INPUT:
line 1: message
line 2: key
Hill Cipher Program Source Code Free
OUTPUT:
line 1: decrypted message
The following is the Playfair Cipher decryption algorithm program in C++.
OUTPUT:
Other cryptography algorithms:
Let us know in the comments if you are having any questions regarding this cryptography cipher Algorithm.
And if you found this post helpful, then please help us by sharing this post with your friends. Thank You